React Hooks, introduced in React 16.8, transformed the way we write components by allowing functional components to manage state, perform side effects, and tap into other React features without needing classes. This shift not only simplifies the component structure but also enhances code reuse and separation of concerns. By "hooking into" React’s state and lifecycle features from functional components, Hooks provide a more direct and concise way to handle component logic, making the code easier to follow, test, and maintain.
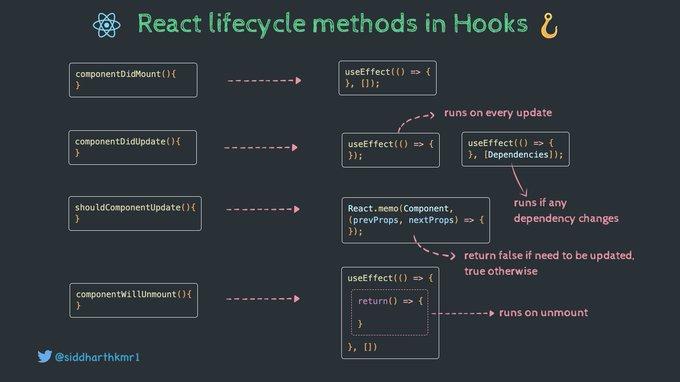
Before React Hooks, functional components were limited in their capabilities, primarily because they couldn’t manage their own state or utilize lifecycle methods. Class components, while powerful, introduced complexities such as understanding the this
keyword, handling bindings, and structuring lifecycle methods correctly. As applications grew, the logic inside class components became harder to manage, leading to issues with code readability and reusability.
Hooks solve common problems like duplicative code and difficulty in sharing logic across components, allowing developers to write cleaner, more modular code. This makes React development more accessible and scalable as applications grow in complexity.
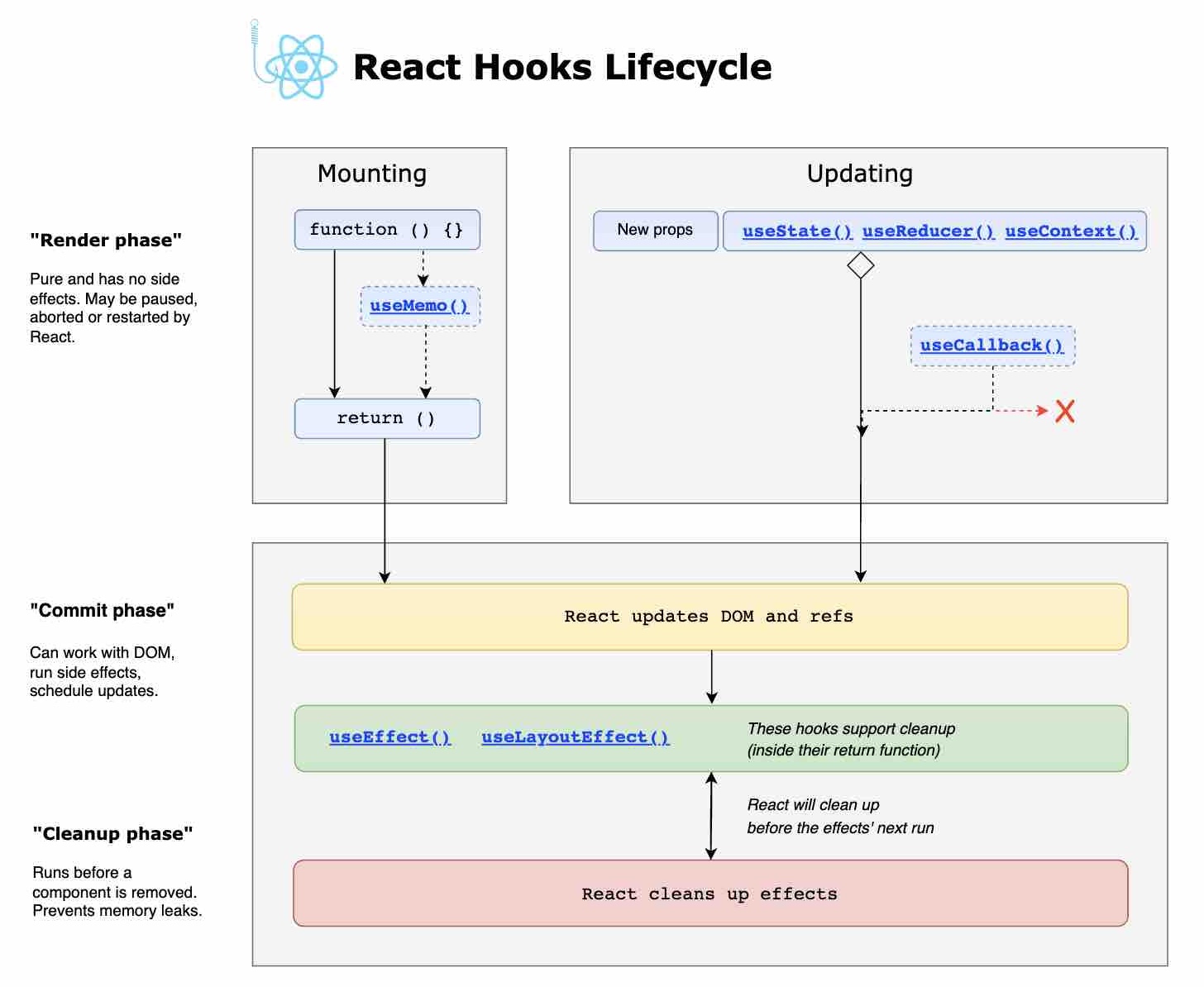
In this article, we will explore what React Hooks are, their significance, good practices, and a comprehensive look at some of the most commonly used hooks.
Commonly used React Hooks
Here are the available React Hooks, along with an in-depth explanation of how each one works and simple code examples:
1. useState
The useState hook is one of the most fundamental and frequently used hooks in React. It allows you to add state to your functional components. Before hooks, state management was only possible in class components. With useState, you can now manage state in a much simpler and more declarative way within functional components.
How it works:
When you call useState, you pass the initial state as an argument, and it returns an array with two values: the current state and a function to update it. Every time you call the update function, React re-renders the component with the new state.
When to Use:
Use useState when you need to manage simple, local state within a functional component. It’s ideal for scenarios where the state is not too complex and doesn’t require intricate updates, such as toggling a boolean, counting, or managing form inputs.
import { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In this example, the useState hook is used to add a state variable count to the Counter component. The hook takes an initial value of 0 as an argument, setting the initial value of count. The useState hook returns an array with two elements: the current value of count and a function setCount to update it. The setCount function updates the count state variable when the button is clicked, and the component re-renders with the new value of count.
2. useEffect
The useEffect hook is used to handle side effects in functional components. Before hooks, side effects like data fetching, subscriptions, or manually changing the DOM were handled in lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount. With useEffect, all these use cases can be managed in one place.
How it works:
useEffect takes two arguments: a function containing the side effect logic, and an optional array of dependencies. The side effect function runs after the component renders. The dependencies determine when the effect should re-run. If the dependencies change, the effect re-runs, if not, it doesn’t.
When to Use:
Use useEffect whenever you need to perform side effects in your component, such as fetching data, subscribing to events, or interacting with the browser's API. It’s also useful for cleaning up resources when the component unmounts or when a dependency changes.
import { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
return (
<div>
<h1>Data:</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
}
In this example, the useEffect hook is used to fetch data from an API when the component mounts.
The useEffect hook takes a function as an argument, which executes after the component renders. This function fetches data from the API and updates the data state variable using the setData function.
The second argument to useEffect is an array of dependencies that controls when the effect should re-run. An empty array means the effect runs only once, upon component mount.
When the component renders, useEffect triggers the API request. Once the response is received, setData updates the data state variable, causing the component to re-render with the new data.
3. useContext
The useContext hook provides a way to access data from a React context directly in a functional component. This hook eliminates the need for wrapping components in the Consumer component when reading context values. It’s particularly useful for sharing state across multiple components without passing props manually at every level.
How it works:
You pass the context object to useContext, and it returns the current value of the context. The hook subscribes to the context, so when the context value changes, the component re-renders with the new value.
When to Use:
Use useContext when you need to consume context values within a component. It’s ideal for scenarios where you need to pass data (like themes, user information, or settings) deeply through the component tree without manually passing props at every level.
ThemeContext.js
import { createContext, useState } from 'react';
const ThemeContext = createContext();
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(theme === 'light' ? 'dark' : 'light');
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
export { ThemeProvider, ThemeContext };
Footer.js
import React, { useContext } from 'react';
import { ThemeContext } from './ThemeContext';
const Footer = () => {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<footer style={{ backgroundColor: theme === 'dark' ? '#333' : '#fff' }}>
<p>Footer</p>
<button onClick={toggleTheme}>Toggle Theme</button>
</footer>
);
};
In this example, the useContext hook is used to access a context object, ThemeContext.
We create ThemeContext using the createContext function from React. A ThemeProvider component wraps the app, providing the theme state and a toggle function through the useState hook. In the Footer component, the useContext hook accesses the theme state and toggleTheme function. The Footer component uses the theme state to style the background color and the toggleTheme function to switch themes when the button is clicked. By using useContext, we can share the theme state between multiple components without having to pass props down manually. This makes it easy to manage global state in our app.
4. useReducer
The useReducer hook is an alternative to useState for managing state in React components, particularly when the state logic is complex or when the next state depends on the previous one. useReducer is ideal for situations where multiple state transitions or actions need to be handled in a predictable and organised manner.
How it works:
useReducer takes two arguments: a reducer function and an initial state. The reducer function accepts the current state and an action, and returns a new state based on the action type. useReducer returns the current state and a dispatch function that you use to trigger state updates.
When to Use:
Use useReducer when your component’s state logic is complex, involves multiple sub-values, or when state transitions depend on various actions. It’s ideal for managing intricate state transitions, like updating a form with multiple inputs, handling complex lists, or implementing features like undo/redo.
import { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>
Increment
</button>
<button onClick={() => dispatch({ type: 'decrement' })}>
Decrement
</button>
</div>
);
}
In this example, the reducer function uses a switch statement to handle actions based on the type property of the action object. If the type is 'increment', the reducer returns a new state with the count property incremented by 1. If the type is 'decrement', it returns a new state with the count property decremented by 1.
In the Counter component, the useReducer hook manages the count property. The dispatch function sends actions to the reducer, which updates the state.
When the user clicks the "Increment" or "Decrement" button, the dispatch function is called with an action object specifying the action. The reducer function processes this action and returns a new state, which updates the component and triggers a re-render.
5. useMemo
The useMemo hook is used to memoize the result of a computation, optimising performance by preventing unnecessary re-computations. It is beneficial in cases where a component performs an expensive calculation or renders a large list, which could slow down the UI if recalculated or re-rendered on every render.
How it works:
useMemo takes two arguments: a function that computes the result, and an array of dependencies. The memoized value is recomputed only when one of the dependencies changes.
When to Use:
Use useMemo when you have expensive calculations or operations that you don’t want to re-compute on every render. It’s particularly useful for derived data that only needs to be recalculated when certain inputs change, such as filtering or sorting a list.
import React, { useState, useMemo } from 'react';
function ExpensiveCalculation({ number }) {
const computeFactorial = (n) => {
console.log('Computing factorial...');
return n <= 1 ? 1 : n * computeFactorial(n - 1);
};
const factorial = useMemo(() => computeFactorial(number), [number]);
return <div>Factorial of {number} is {factorial}</div>;
}
In this example, the ExpensiveCalculation component receives a number prop and calculates its factorial using the computeFactorial function, which is a recursive function for computing factorial values.
The useMemo hook is used to memoize the result of the computeFactorial function. It takes a function as its first argument to compute the memoized value—in this case, the computeFactorial function.
The second argument to useMemo is an array of dependencies, which determines when the memoized value should be recalculated. Here, the dependency is the number prop.
6. useCallback
The useCallback hook is used to memoize a function, ensuring that the function reference remains stable between re-renders. This is especially useful when passing callback functions to child components that rely on reference equality to prevent unnecessary re-renders. Without useCallback, a new function instance would be created on every render, potentially causing performance issues or unexpected behaviour in child components.
How it works:
useCallback takes two arguments: the callback function itself and an array of dependencies. The callback is only recreated if one of the dependencies changes. If the dependencies do not change, the same function instance is returned, which prevents child components from re-rendering unnecessarily.
When to Use:
Use useCallback when you are passing functions as props to child components, particularly if those components rely on reference equality to determine if they should re-render. It’s also useful when dealing with expensive computations within functions that you don’t want to recalculate on every render.
import React, { useState, useCallback } from 'react';
function ExpensiveComponent({ onCalculate }) {
console.log('ExpensiveComponent rendered');
return <button onClick={onCalculate}>Calculate</button>;
}
function ParentComponent() {
const [count, setCount] = useState(0);
const handleCalculate = useCallback(() => {
console.log('Calculation performed');
}, []);
return (
<div>
<p>Count: {count}</p>
<ExpensiveComponent onCalculate={handleCalculate} />
<button onClick={() => setCount(count + 1)}>Increment Count</button>
</div>
);
}
In this example, the handleCalculate function is passed to the ExpensiveComponent as a prop. Without useCallback, handleCalculate would be recreated on every render of ParentComponent, potentially causing ExpensiveComponent to re-render unnecessarily. By wrapping handleCalculate in useCallback with an empty dependency array, the function reference remains stable unless explicitly changed.
Good Practices for Using React Hooks
To make the most of React Hooks and ensure your components are efficient and maintainable, follow these best practices:
- Use Hooks at the Top Level: Always call hooks at the top level of your component or custom hook. This ensures that hooks are called in the same order on every render, which is crucial for maintaining consistent behaviour .
- Avoid Conditional Hook Calls: Never call hooks inside conditions, loops, or nested functions. This can lead to unpredictable behaviour and violates the rules of hooks.
- Specify Dependencies Correctly: When using useEffect, useCallback, or useMemo, provide a correct and complete list of dependencies. Omitting dependencies or including unnecessary ones can lead to stale data or excessive re-renders.
- Use Custom Hooks for Reusable Logic: Extract common logic into custom hooks. This promotes reusability and helps keep your components focused on rendering UI.
- Optimize Performance with useMemo and useCallback: Use useMemo to memoize expensive calculations and useCallback to memoize callback functions. This can help prevent unnecessary re-renders and improve performance.
- Manage State Updates Carefully: Be mindful of how state updates are handled. For example, when using useState, ensure that state updates are applied correctly, especially when state depends on previous values.
- Use Context Wisely: When using useContext, ensure that context values are stable and consider memoizing the context provider’s value to avoid unnecessary re-renders of consumer components.
- Keep Hooks Simple: Custom hooks should be kept simple and focused on a single piece of functionality. Complex logic should be broken down into smaller, manageable hooks.
Summary
React Hooks have transformed how we build components by enabling state management, side effects, and context handling directly within functional components. This shift simplifies code structure, improves readability, and promotes better code reuse.
In this blog, we've covered the essential Hooks—useState, useEffect, useContext, useReducer, useMemo, and useCallback—highlighting their purposes and use cases. By adhering to good practices, such as managing dependencies correctly and using custom Hooks for reusable logic, you can enhance both performance and maintainability in your React applications.
Embracing React Hooks allows you to write cleaner, more efficient code and build more scalable and maintainable applications with ease.