Remember the days when your API would return a massive JSON payload, but all you needed was a user's name and profile picture? Or when you'd have to make three separate API calls just to get related data that logically belongs together? If you're nodding your head, you're not alone—and that's exactly why GraphQL exists.
Born out of necessity at Facebook in 2012, GraphQL was created to solve real problems the company faced while rebuilding their mobile applications. As Facebook's mobile presence grew, they discovered the limitations of their REST APIs: inflexible endpoints that either returned too much data (slowing down mobile apps) or too little (requiring multiple round-trips to the server).
"We were frustrated with the differences between the data we wanted to use in our apps and the server queries they required. We don't think of data in terms of resource URLs, secondary keys, or join tables; we think about it in terms of a graph of objects and the models we ultimately use in our apps." — Lee Byron, GraphQL co-creator
At its core, GraphQL flips the traditional API model on its head. Instead of the server dictating what data you get, the client specifies exactly what it needs. Think of it as ordering à la carte instead of accepting a fixed menu—you get precisely what you want, nothing more, nothing less.
Why GraphQL vs. REST APIs?
REST has been the standard for API development for years, so why switch? Here are some compelling reasons:
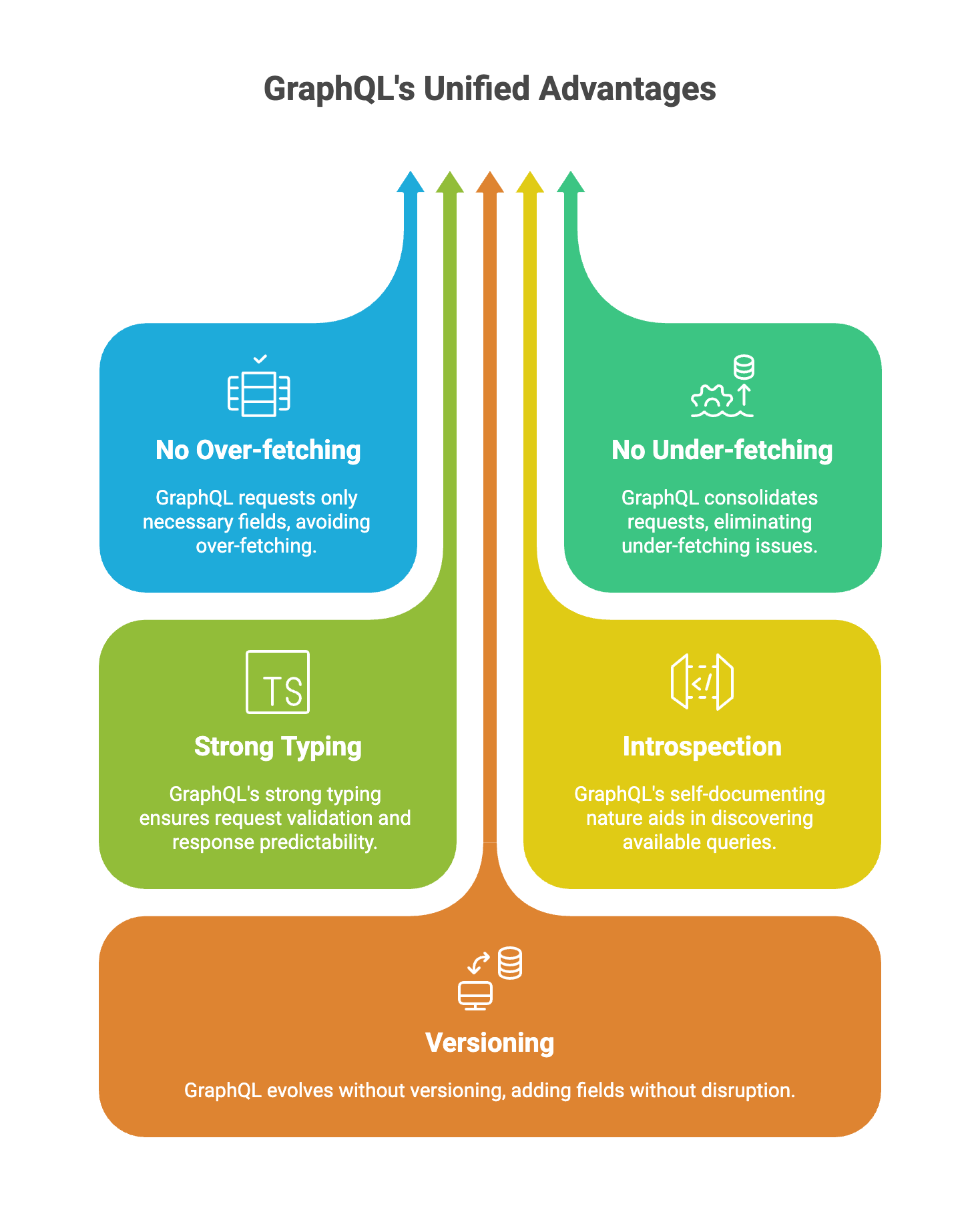
Before we dive deeper, I'll share a quick story. On one of my projects, we were building a dashboard that displayed data from six different REST endpoints. Page load was frustratingly slow because we had to wait for all requests to complete sequentially. After migrating to GraphQL, we consolidated those six requests into one precise query—cutting load time by 70% and making our UX team very happy. That's the kind of real-world impact GraphQL can have.
When to Choose GraphQL for Your Project
Not every project needs GraphQL. Understanding when to use it can save you time and improve your application architecture.
Ideal Use Cases for GraphQL
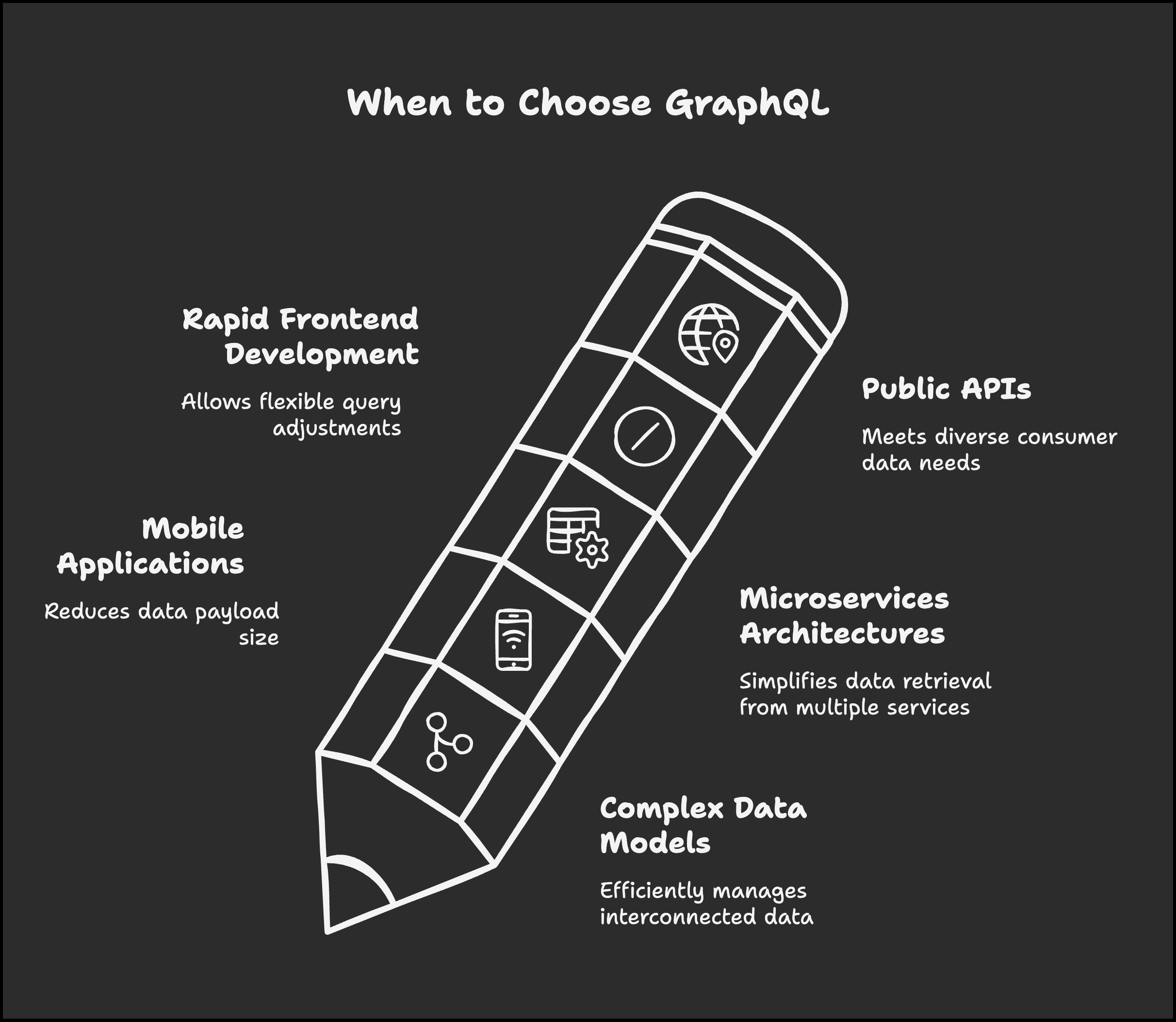
When to Stick with REST
.png)
Industry Adoption
Major companies across various industries have embraced GraphQL:
- Tech: GitHub, Twitter, Shopify, Airbnb
- E-commerce: Walmart, eBay, Etsy
- Media: The New York Times, Netflix, Spotify
- Finance: PayPal, Intuit
- Travel: Expedia, Airbnb
According to the State of JavaScript 2023 survey, GraphQL usage among developers has grown by 24% year-over-year, showing strong industry momentum.
💡 Pro Tip: When evaluating GraphQL for your project, start by mapping out your data requirements and API consumption patterns. If you find yourself creating many specialized REST endpoints or dealing with over-fetching problems, that's a strong indicator that GraphQL would be beneficial.
Core Concepts
Let's break down the key building blocks that make GraphQL tick before we dive into implementation.
The Schema: Your API's Contract
The schema is the foundation of any GraphQL API—it's a strongly typed description of your entire API's capabilities. Think of it as a contract between your server and client, defining what queries are possible and what data structures to expect.
Type System
GraphQL's type system gives you the tools to model complex domains:
- Object Types: Define the entities in your API (like Book, Author, User)
- Scalar Types: The primitives—
String
,Int
,Float
,Boolean
, andID
- Enums: For when you need a specific set of allowed values
- Lists and Non-Nulls: Indicated by
[Type]
andType!
respectively - Input Types: Special objects used for passing complex arguments
Operation Types
GraphQL supports three types of operations:
- Queries: For fetching data (similar to GET in REST)
- Mutations: For modifying data (similar to POST/PUT/DELETE in REST)
- Subscriptions: For real-time updates via persistent connections
💡 Pro Tip: Think of queries, mutations, and subscriptions as the "GET," "POST/PUT/DELETE," and "WebSocket" of the GraphQL world, respectively.
Building a Book Library API: A Practical Example
Let's put theory into practice by building a simple book library API. This example will demonstrate all the core concepts of GraphQL in action.
Setting Up Your GraphQL Server
First, let's set up the tools we need:
npm init -y
npm install apollo-server-express express graphql
Now, create a file named index.js:
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
// We'll define our schema and resolvers here
async function startServer() {
// Create Express app
const app = express();
// Create Apollo Server
const server = new ApolloServer({ typeDefs, resolvers });
await server.start();
// Apply middleware
server.applyMiddleware({ app });
// Start the server
app.listen({ port: 4000 }, () =>
console.log(`Server ready at http://localhost:4000${server.graphqlPath}`)
);
}
startServer();
Designing the Schema
Our book library API will need to track books and authors. Here's how we define that in GraphQL:
// Define our schema
const typeDefs = gql`
"""
Author of books in our library
"""
type Author {
id: ID!
name: String!
bio: String
books: [Book!]!
}
"""
Book in our library collection
"""
type Book {
id: ID!
title: String!
summary: String
publishedYear: Int
genre: String
author: Author!
}
"""
Queries available in our API
"""
type Query {
books: [Book!]!
book(id: ID!): Book
authors: [Author!]!
author(id: ID!): Author
booksByGenre(genre: String!): [Book!]!
}
"""
Mutations for modifying data
"""
type Mutation {
addBook(title: String!, authorId: ID!, summary: String, publishedYear: Int, genre: String): Book!
addAuthor(name: String!, bio: String): Author!
}
`;
In this schema:
- The
!
symbol marks fields as non-nullable (the server must always provide a value) [Book!]!
means "a non-nullable array of non-nullable Book objects"- Each type has a clear purpose and relationships to other types
- The schema is self-documenting with clear descriptions
Implementing Resolvers
Resolvers connect our schema to actual data. For this example, we'll use in-memory data, but in a real application, you would connect to a database or external API:
// Sample data
const authors = [
{ id: '1', name: 'J.K. Rowling', bio: 'British author best known for the Harry Potter series' },
{ id: '2', name: 'George Orwell', bio: 'English novelist known for dystopian fiction' }
];
const books = [
{ id: '1', title: 'Harry Potter', authorId: '1', publishedYear: 1997, genre: 'Fantasy', summary: 'A young wizard discovers his heritage' },
{ id: '2', title: '1984', authorId: '2', publishedYear: 1949, genre: 'Dystopian', summary: 'A man rebels against a totalitarian regime' }
];
// Resolvers
const resolvers = {
// Field resolvers for Query type
Query: {
books: () => books,
book: (_, { id }) => books.find(book => book.id === id),
authors: () => authors,
author: (_, { id }) => authors.find(author => author.id === id),
booksByGenre: (_, { genre }) => books.filter(book => book.genre === genre)
},
// Field resolvers for Book type
Book: {
author: (book) => authors.find(author => author.id === book.authorId)
},
// Field resolvers for Author type
Author: {
books: (author) => books.filter(book => book.authorId === author.id)
},
// Field resolvers for Mutation type
Mutation: {
addBook: (_, { title, authorId, summary, publishedYear, genre }) => {
const newBook = {
id: String(books.length + 1),
title,
authorId,
summary,
publishedYear,
genre
};
books.push(newBook);
return newBook;
},
addAuthor: (_, { name, bio }) => {
const newAuthor = {
id: String(authors.length + 1),
name,
bio
};
authors.push(newAuthor);
return newAuthor;
}
}
};
When I first implemented resolvers like these, I was amazed at how naturally they mapped to my data model. Notice how the resolver structure mirrors our schema? That's one of GraphQL's most elegant aspects—the API design feels intuitive and natural.
Interacting with Our API
Now that our server is set up, let's explore how to interact with it through queries and mutations.
Basic Query: Getting All Books
query GetAllBooks {
books {
title
genre
publishedYear
summary
author {
name
bio
}
}
}
This query fetches all books with their titles, genres, published years, summaries, and author details.
Field Selection: Getting Only What You Need
query GetBookTitles {
books {
title
publishedYear
# We're not requesting other fields we don't need
}
}
This demonstrates the power of GraphQL—we're only requesting the specific fields we need, reducing the payload size.
Filtering with Arguments
query GetFantasyBooks {
booksByGenre(genre: "Fantasy") {
title
author {
name
}
}
}
This query filters books by genre and returns only their titles and author names.
Using Aliases for Multiple Queries
query GetTwoBooks {
harryPotter: book(id: "1") {
title
author {
name
}
}
nineteenEightyFour: book(id: "2") {
title
author {
name
}
}
}
With aliases, we can request multiple resources in a single query and give them descriptive names in the response.
Reusing Fields with Fragments
fragment BookDetails on Book {
title
publishedYear
author {
name
}
}
query BooksWithFragment {
book(id: "1") {
...BookDetails
}
books {
...BookDetails
}
}
Fragments let us reuse selections of fields, making our queries more maintainable.
Creating Data with Mutations
mutation AddNewBook {
addBook(
title: "The Great Gatsby"
authorId: "2"
publishedYear: 1925
genre: "Classic"
summary: "A story of wealth, love, and the American Dream"
) {
id
title
}
}
This mutation adds a new book and returns its ID and title.
On a recent project, we implemented a similar API for a digital library. The front-end developers particularly loved being able to experiment with the API using GraphQL's built-in IDE (like GraphiQL or Apollo Sandbox). They could explore the schema and test queries without having to reference external documentation constantly.
GraphQL Good Practices
Based on my experience building GraphQL APIs, here are some best practices to follow:
Schema Design
- Follow naming conventions: Use
camelCase
for fields and arguments,PascalCase
for types - Be descriptive but concise: Names should clearly convey purpose without being overly verbose
- Design with the consumer in mind: Model your schema around how the data will be used, not how it's stored
- Use custom scalars wisely: For specialized data types like
Date
orEmail
to enhance validation
💡 Pro Tip: Write schema descriptions (using """ multi-line comments """) for all types and fields. These show up in GraphQL tools and make your API self-documenting.
Performance Optimization
- Implement dataloaders: Use DataLoader to batch and cache database queries, solving the N+1 query problem
- Add complexity limits: Protect your server by limiting query depth and complexity
- Use pagination: For large collections, implement cursor-based pagination using the Relay Connection spec
- Consider persisted queries: In production, use persisted queries to reduce request size and improve security
Here's a simple DataLoader implementation example:
const DataLoader = require('dataloader');
// Create a loader for authors
const authorLoader = new DataLoader(authorIds => {
return Promise.all(
authorIds.map(id => authors.find(author => author.id === id))
);
});
// Use in resolver
const resolvers = {
Book: {
author: (book) => authorLoader.load(book.authorId)
}
};
Common Pitfalls to Avoid
- Over-nesting relations: Deeply nested relations can cause performance issues
- Ignoring nullability: Be intentional about which fields can return null
- Exposing implementation details: Your schema should represent your domain, not your database structure
- Neglecting error handling: Implement proper error handling in resolvers
Popular GraphQL Client Libraries
To effectively consume GraphQL APIs from your applications, consider these popular client libraries:
React Applications
- Apollo Client: Comprehensive state management with caching
- Relay: Facebook's GraphQL client with performance optimizations
- URQL: Lightweight alternative with a focus on simplicity
Mobile Development
- Apollo iOS/Android: Apollo Client for mobile platforms
- GraphQL iOS/Android: Lighter-weight alternatives
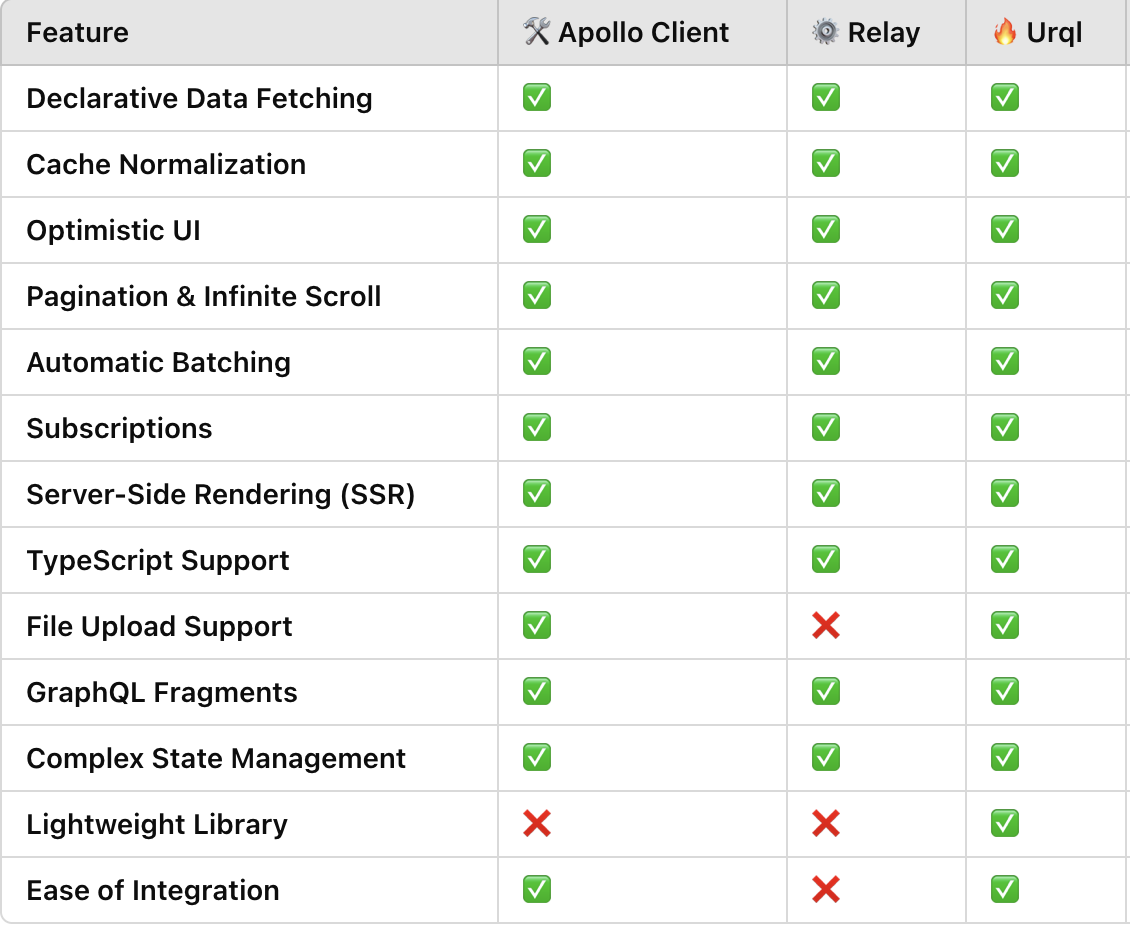
Conclusion
We've covered the fundamentals of GraphQL—from understanding its core philosophy to building a working book library API. What started as Facebook's internal solution has grown into an industry-changing approach to API development.
The beauty of GraphQL lies in its client-centric approach. By giving clients the power to ask for exactly what they need, we build more efficient, flexible, and maintainable applications.
In my experience, teams that adopt GraphQL typically see:
- Frontend developers gaining independence to iterate without waiting for API changes
- Backend developers focusing on defining capabilities rather than specific endpoints
- Mobile apps performing better with reduced payload sizes
- Documentation staying current thanks to the self-documenting nature of the schema
This blog post is just the beginning of your GraphQL journey. In our next post, "Automatic Schema Generation with PostgreSQL and PostGraphile," we'll dive into connecting your GraphQL API to a PostgreSQL database using DBMate for migrations.
Have you implemented GraphQL in your projects? What challenges did you face? I'd love to hear about your experiences in the comments below!
The code examples in this post are simplified for clarity and educational purposes. In a production environment, you'd want to add proper error handling, authentication, and data persistence.